Play Go Fish Online Against Computer
- Play Go Fish Online Against Computer
- How To Play Go Fish
- Play Go Fish Online Against Computer Game
- Play Go Fish Card Game
- Play Go Fish Online Against Computer Online
Backgammon is the 28th game we make here at CardGames.io. It's been requested a lot over the years, but the main reason it was never created was the custom graphics needed for it. Well, finally we did it! Backgammon is the first game developed by CardGames.io's two new full.
- Try playing an online chess game against a top chess computer. You can set the level from 1 to 10, from easy to grandmaster. If you get stuck, use a hint or take back the move. When you are ready to play games with human players, register for a free Chess.com account!
- Leave it at that, and click the OK button. Now you can click on the game panel (in this case, Euchre), and go straight to a table where only robots are allowed. The game will start immediately with 'robots'! You are now playing against the computer. Don't forget to uncheck the 'Private Table' option if you want to go back to playing with people.
Go Fish game
For this assignment, you will write a program that allows one player to play a game of Go Fish against the computer. Go Fish is a game that uses a standard deck of 52 cards. Specifically, each card has a rank and a suit. There are 13 ranks: the numbers 2 through 10, Jack (usually represented with the letter J), Queen (Q), King (K), and Ace (A). There are 4 suits: clubs, diamonds, hearts, and spades. In a 52-card deck, there is one card of each rank for each suit.
A game of Go Fish between two players proceeds as follows:
* The deck of cards is shuffled, randomizing the order of the cards.
* Each player is dealt 7 cards.
* The remaining cards are placed face-down (i.e. with their rank and suit hidden) on the table to form the 'stock'.
* One of the players (player A) begins the game by asking the other player (player B) for all of his/her cards of a specific rank (e.g. 'Please give me all of your 7's'). To ask for a given rank, a player must have at least one card of that rank in his/her hand.
* If player B has cards of the requested rank, he/she must give *all* of his/her cards of that rank to player A, and player A gets to take another turn.
* If player B does not have any cards of the requested rank, he/she says 'Go fish', and player A must select one card from the stock. If that card has the rank player A originally requested, then player A gets to take another turn. Otherwise, it becomes player B's turn.
* If at any point a player runs out of cards, then, when it is that player's turn to play, they may draw a card from the stock and ask for cards of that rank. If a player runs out of other cards when it is the other player's turn to ask for a rank, the other player may continue to ask for a rank and draw from the stock until they draw a rank other than the one they asked for.
* If a player collects all four cards of the same rank, this is called a 'book', and the player lays down his/her book on the table.
* The game continues with the players alternating turns until all of the books are laid down. At the end of the game, the player with the most books wins.
Needed classes
To write your Go Fish game, you should implement the following classes, including the specified members and methods. You may also add more members and methods, as needed.
```
class Card {
private:
int rank; // Should be in the range 0-12.
int suit; // Should be in the range 0-3.
public:
// must have constructors, destructor, accessor methods, and mutator methods
};
```
In the `Card` class above, `rank` and `suit` are represented with `int` values, but you must also have some way to map those values to representations players will be familiar with (e.g. a string representation of the suit or rank).
```
class Deck {
private:
Card cards[52];
int n_cards; // Number of cards remaining in the deck.
public:
// must have constructors, destructor, accessor methods, and mutator methods
};
```
The `Deck` class is the source of all of the cards. Cards will initially start in a `Deck` object and then be transferred to players' hands. An important method that should be implemented for the `Deck` class is one to remove a card and return it so it can be placed in a player's hand.
```
class Hand {
private:
Card* cards;
int n_cards; // Number of cards in the hand.
public:
// must have constructors, destructor, accessor methods, and mutator methods
};
```
The `Hand` class will hold the cards in one player's hand. The number of cards a player holds may change, so the size of the array of `Card` objects in a hand may also need to change. Cards may be added to a player's hand and removed from a player's hand, so the `Hand` class will need functions to do both of those things. Other useful methods might check for a book and remove a book from the player's hand.
```
class Player {
private:
Hand hand;
Play Go Fish Online Against Computer
int* books; // Array with ranks for which the player has books.int n_books;
public:
// must have constructors, destructor, accessor methods, and mutator methods
};
```
The `Player` class represents a single player. Each `Player` will have a `Hand` object representing its hand and an array keeping track of the books the player has laid down. Depending on how you implement things, the `Player` class may need methods to add and remove cards from their hand or to check their hand for books. Another useful method the `Player` class might have is one to figure out what rank they want to ask for. Note that the `Player` class must represent both the human player and the computer player. You should write your class methods accordingly and add any extra data members needed to accomplish this.
```
class Game {
private:
Deck cards;
Player players[2];
public:
// must have constructors, destructor, accessor methods, and mutator methods
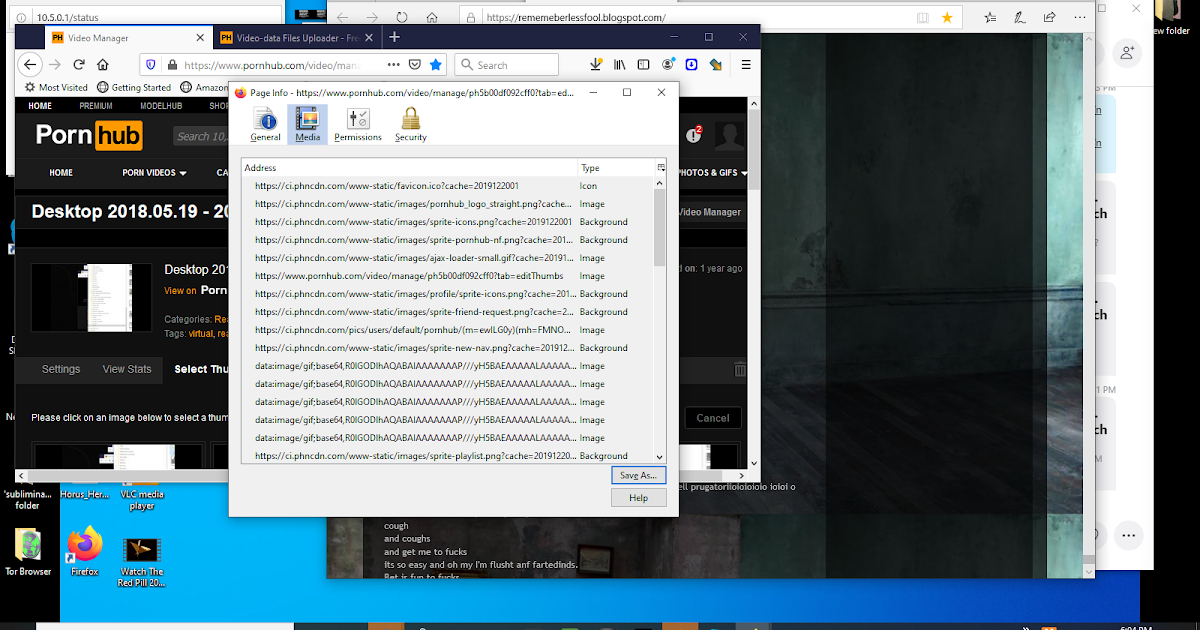
```
The `Game` class represents the state of an entire game. It contains objects representing the deck of cards and both players. It would be useful to have methods in the `Game` class that check whether the game is over and that execute a player's turn.
Program features
Your program should do the following:
* Set up a deck of 52 cards as described above.
* Shuffle the deck (i.e. randomize the order of cards).
* Deal the cards to the two players.
* Play a game of Go Fish as described above, with the following gameplay features:
* Print the current state of the game (e.g. the cards held by the human player and the books laid down by both the human player and the computer) after each turn (keeping the computer player's cards hidden).
* On the human player's turn, prompt the user for a rank to ask for. When they enter a rank, either give all of the computer player's cards of that rank to the human (if the computer player has cards of the requested rank) or execute a 'go fish', drawing a card from the stock into the human player's hand. Make sure to alert the user what the result of their turn is.
* On the computer player's turn, the computer should randomly select a rank from its hand for which to ask the other player, and the appropriate actions should be taken (i.e. cards are taken from the human player's hand, or the computer draws a card from the stock). The user should be alerted about what the computer player asked for and what the result of its turn was.
* Once the game is over, you should announce the winner and ask the user if they want to play again.
* You should not have any memory leaks in your program.
Program design
It is up to you how to design an interface to allow the user to play the Go Fish game.
Your program should use the classes above along with any other classes or functions you need. Your program should be well modularized. That is, it should be factored into reasonably-sized (preferably small) functions that each perform some specific logical task.
You should separate your source code several files:
* `gofish.cpp` - This should contain your `main()` function.
* You should also have one interface header (`.hpp`) file and one implementation (`.cpp`) file for each of the classes above, as well as for any additional classes you implement.
You should also write a `Makefile` that specifies the compilation of your program.
In this tutorial you will learn how to generate unlimited resources for Go Fish 1.24 in a few minutes. Try the version that suits you and download it below. Make your gaming experience easier by using Go Fish 1.24 generator.
Generators are in trend these days when you think about games. Why? Due to the facts that this type of apps gives you permission to play Go Fish 1.24 with unlimited resources and it’s saves your money for more important things.
Using Go Fish 1.24 hack has got more than one advantage during the game. Firstly, you may now have unlimited resources for Go Fish 1.24 and zero ads. It is valid for online servers, so this means that you will receive everything in the Go Fish 1.24 game, in which you are having so much fun with right now.
Secondly, do you want free resources for Go Fish 1.24? Using our Go Fish 1.24 hack you can have as many resources as you wish from shop and rank the first position in leaderboard.
About Go Fish 1.24 Game:
Play the popular and exciting card game classic, Go Fish! A card game for kids and grown-ups of all ages! The object of this single-player Go Fish card game is to form the most pairs of cards. Play against a gang of hilarious computer-controlled opponents. Compare your point totals with other card players around the world in the worldwide Go Fish leaderboard!The game features:- Single Game Mode: A simple game where you can select your computer opponent.- Career Mode: Your wins and losses are counted and stored for the Go Fish leaderboard.- Onscreen to tips to make the Go Fish card game easy to learn for kids.- Unlockable characters with fun dialogue
Game Generator for Go Fish 1.24
There are many ways to hack on the web but we can guarantee you that not even one of them rises to the performance provided by RealGenerator.net
While choosing to hack Go Fish 1.24 you need to take into consideration the following things which are completely essential:
Go Fish 1.24 Generator no root or jailbeak:
A lot of hacks require a rooted android device which leads to many headaches and in some cases locks your phone. Using RealGenerator you can generate limitless resources for Go Fish 1.24 from your desktop, android or iOS device with no root or jailbreak required.
Go Fish 1.24 Generator for Desktop:
RealGenerator developers offers you for free two versions of the application, one of these is the desktop variant which runs online without download required.
UPDATE: At the moment, we are working on a better version for the desktop and we kindly ask you to download and use Go Fish 1.24 hack for android or iOS if you would like to generate unlimited resources for Go Fish 1.24.
Go Fish 1.24 Generator for Android:
If we relate to the needs of the players, the best hack of the moment is RealGenerator.apk.
Go Fish 1.24 for iOS:
Due to the huge amount of requests, our team developed RealGenerator for iOS users.
Download Go Fish 1.24 hack for iOS from below.
Go Fish 1.24 hack updated:
As a result of the large number of active players, the games are updated very often. Due to this, some cheats sometimes work only for a short while.
RealGenerator is linked to the games database via Google Play and App Store which means that it will be possible to crack the game even if it has periodical updates.
Safe and undetectable hack for Go Fish 1.24
No matter if you choose the mobile version or desktop one, RealGenerator it’s secure and untraceable.
The coding of our mobile version tool is done by the best code writers and this is why your account is safe when you use Go Fish 1.24 hack provided by RealGenerator.
Step 1: Download RealGenerator.apk using below link
Step 2: Install RealGenerator on your android device;
Troubleshooting:
Step 2.1: If the installation is blocked, go to settings menu;
Step 2.2: Enable the installation from unknown sources (“Allow installation of apps from sources other than the Play Store”).
Step 3: Open up Real Generator, search for Go Fish 1.24 and press “Get Started Now”;
Step 4: Enter your username (or Google Play e-mail associated with Go Fish 1.24 account) and the amount of free resources. Press “GENERATE NOW” button;
Step 4.1: If you have problems with the translation, our .apk comes with preinstalled Google Translate feature. Just select your language from menu.
Step 5: You’re done, open Go Fish 1.24 and use your benefits as you want.
Must read:
How To Play Go Fish
Text fragments and photos from this tutorial are used as a general example. Due to amount of hacks provided we need a lot of space for images this is why we use same pictures.
Step 1: Download the RealGenerator iOS version from below;
Step 2: Find RealGenerator in Files > Downloads;
Step 3: Access “Profile Downloaded” from Settings menu;
Play Go Fish Online Against Computer Game
Step 4: Select RealGenerator app, press “Install”, accept Consent, enter PIN and press “Done”. RealGenerator doesn’t need special permissions like other apps, in these days this is rare!
Step 5: Open RealGenerator and select Go Fish 1.24 from list;
Step 6: Enter username or iCloud account associated with Go Fish 1.24 and press “GENERATE NOW” button;
Step 7: You’re done! Open Go Fish 1.24 and spend your resources as you want.
Play Go Fish Card Game
That is all tutorial about How to Hack Go Fish 1.24 with RealGenerator, simple and organized as I said. If you have any problems, we kindly ask you to use our contact form, comment section or write us on social media platforms.
Play Go Fish Online Against Computer Online
Download Links Updated: